Table of Contents
Sell anything on your social platform
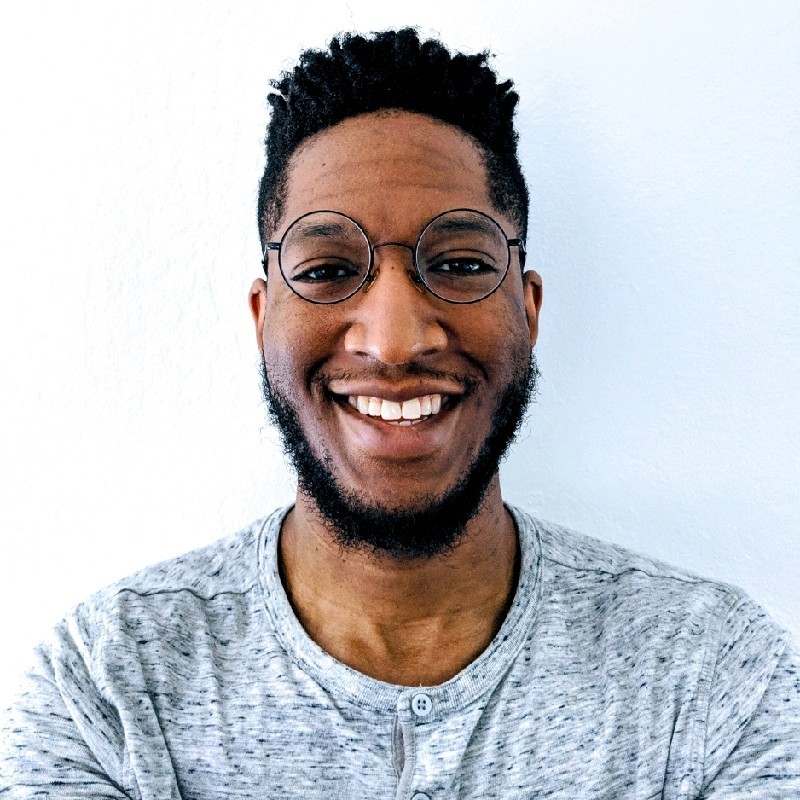
Ajibade Adebayo
Software Engineer @ Rye
Nov 8, 2023
4 min read
Learn how to effectively sell products on social platforms with our comprehensive guide. Discover strategies for leveraging social media to boost sales, engage with customers, and grow your business online.
The problem
Building and scaling eCommerce to sell any products is often a costly and ambitious goal for most social platforms without massive clout like TikTok or marketplace infrastructures like Meta.
Building the rudimentary order processing and cart technology is not hard. What is difficult - is acquiring merchants, orchestrating order placement, payment processing, building a consistent user experience, and consolidating data across platforms. Social platforms end up spending millions to solve the series of issues.
This guide shows you how you can skip all of that work.
One of the challenges involves acquiring merchants in order to fetch their up-to-date product data for your inventory, place orders programmatically to make sure they are fulfilled, and to get affiliate commissions on sales. For instance, a creator recommends 4 products for a winter skincare routine in a video. The products are from 4 different merchants, some listed on Amazon Business, some Shopify. To enable users purchase them all in one go, you must tackle:
User information validation (shipping address, billing address, phone validation, etc.)
Fraudulent payment issues
3DS payment verifications
Issues with third-party logistics (3PL)
Building and scaling eCommerce to sell any products is often a costly and ambitious goal for most social platforms without massive clout like TikTok or marketplace infrastructures like Meta.
Building the rudimentary order processing and cart technology is not hard. What is difficult - is acquiring merchants, orchestrating order placement, payment processing, building a consistent user experience, and consolidating data across platforms. Social platforms end up spending millions to solve the series of issues.
This guide shows you how you can skip all of that work.
One of the challenges involves acquiring merchants in order to fetch their up-to-date product data for your inventory, place orders programmatically to make sure they are fulfilled, and to get affiliate commissions on sales. For instance, a creator recommends 4 products for a winter skincare routine in a video. The products are from 4 different merchants, some listed on Amazon Business, some Shopify. To enable users purchase them all in one go, you must tackle:
User information validation (shipping address, billing address, phone validation, etc.)
Fraudulent payment issues
3DS payment verifications
Issues with third-party logistics (3PL)
Building and scaling eCommerce to sell any products is often a costly and ambitious goal for most social platforms without massive clout like TikTok or marketplace infrastructures like Meta.
Building the rudimentary order processing and cart technology is not hard. What is difficult - is acquiring merchants, orchestrating order placement, payment processing, building a consistent user experience, and consolidating data across platforms. Social platforms end up spending millions to solve the series of issues.
This guide shows you how you can skip all of that work.
One of the challenges involves acquiring merchants in order to fetch their up-to-date product data for your inventory, place orders programmatically to make sure they are fulfilled, and to get affiliate commissions on sales. For instance, a creator recommends 4 products for a winter skincare routine in a video. The products are from 4 different merchants, some listed on Amazon Business, some Shopify. To enable users purchase them all in one go, you must tackle:
User information validation (shipping address, billing address, phone validation, etc.)
Fraudulent payment issues
3DS payment verifications
Issues with third-party logistics (3PL)
The solution
This is where Rye’s Sell Anything API comes to play - an integration that solves for the above problems (and many more). This includes the ability for you to list and sell any products listed on Amazon or Shopify without having to build 1:1 merchant relationships. Our users simply plug into our APIs to build a complete end-to-end eCommerce experience in 7 steps. These are the fundamental blocks extensible to marketplace and gifting platforms as well.
Listing products to sell
Place order for products selected by user
Post-checkout
Pre-requisites
This tutorial enables you to add products into your platform’s own inventory, for users to select and add products to cart, submit the cart order, and for you to keep track of orders (using web-hooks).
We will be:
Using JavaScript as our primary language
Using the
ApolloClient
as our GQL client for making the API calls
You need your Rye API Key headers and Payment Gateway Headers for this tutorial. Create an account or login to the Rye console and go to Account
This tutorial enables you to add products into your platform’s own inventory, for users to select and add products to cart, submit the cart order, and for you to keep track of orders (using web-hooks).
We will be:
Using JavaScript as our primary language
Using the
ApolloClient
as our GQL client for making the API calls
You need your Rye API Key headers and Payment Gateway Headers for this tutorial. Create an account or login to the Rye console and go to Account
This tutorial enables you to add products into your platform’s own inventory, for users to select and add products to cart, submit the cart order, and for you to keep track of orders (using web-hooks).
We will be:
Using JavaScript as our primary language
Using the
ApolloClient
as our GQL client for making the API calls
You need your Rye API Key headers and Payment Gateway Headers for this tutorial. Create an account or login to the Rye console and go to Account
Step 0: Initialize GQL (graph query language) client via API key headers
import { ApolloClient, InMemoryCache } from '@apollo/client';
export const apolloClient = new ApolloClient({
uri: "https://graphql.api.rye.com/v1/query",
cache: new InMemoryCache(),
connectToDevTools: true,
headers: <YOUR_API_KEY_HEADERS>
import { ApolloClient, InMemoryCache } from '@apollo/client';
export const apolloClient = new ApolloClient({
uri: "https://graphql.api.rye.com/v1/query",
cache: new InMemoryCache(),
connectToDevTools: true,
headers: <YOUR_API_KEY_HEADERS>
import { ApolloClient, InMemoryCache } from '@apollo/client';
export const apolloClient = new ApolloClient({
uri: "https://graphql.api.rye.com/v1/query",
cache: new InMemoryCache(),
connectToDevTools: true,
headers: <YOUR_API_KEY_HEADERS>
Step 1: Add products you want to sell to Rye’s inventory
Some products on Amazon Business or Shopify may not be logged in the Rye inventory. Products can be added to our inventory by the requestProductByURL
mutation.
Input: The URL for the product
Output: The ID of the product
import { gql, useMutation } from "@apollo/client";
const REQUEST_PRODUCT = gql`
mutation RequestProductByURL($input: RequestProductByURLInput!) {
requestProductByURL(input: $input) {
productID
}
}
`;
const [requestProduct, { data: requestProductData, loading: loadingProductData }] = useMutation(REQUEST_PRODUCT);
const onRequestProduct = () => {
const input = {
url: productUrl,
marketplace: "SHOPIFY",
};
requestProduct({ variables: { input }})
};
Some products on Amazon Business or Shopify may not be logged in the Rye inventory. Products can be added to our inventory by the requestProductByURL
mutation.
Input: The URL for the product
Output: The ID of the product
import { gql, useMutation } from "@apollo/client";
const REQUEST_PRODUCT = gql`
mutation RequestProductByURL($input: RequestProductByURLInput!) {
requestProductByURL(input: $input) {
productID
}
}
`;
const [requestProduct, { data: requestProductData, loading: loadingProductData }] = useMutation(REQUEST_PRODUCT);
const onRequestProduct = () => {
const input = {
url: productUrl,
marketplace: "SHOPIFY",
};
requestProduct({ variables: { input }})
};
Some products on Amazon Business or Shopify may not be logged in the Rye inventory. Products can be added to our inventory by the requestProductByURL
mutation.
Input: The URL for the product
Output: The ID of the product
import { gql, useMutation } from "@apollo/client";
const REQUEST_PRODUCT = gql`
mutation RequestProductByURL($input: RequestProductByURLInput!) {
requestProductByURL(input: $input) {
productID
}
}
`;
const [requestProduct, { data: requestProductData, loading: loadingProductData }] = useMutation(REQUEST_PRODUCT);
const onRequestProduct = () => {
const input = {
url: productUrl,
marketplace: "SHOPIFY",
};
requestProduct({ variables: { input }})
};
Step 2: Fetch product data from Rye’s inventory
Now that the product is in Rye’s inventory, you can fetch product information via the productByID
query. This allows you to showcase products and display accurate, up-to-date information, and allows users to make informed purchasing decisions.
Input:
The product ID
The marketplace of the product ('Amazon' or 'Shopify')
Output:
All the requested product data
The code snippet below only fetches specific product data including title, vendor, variants, and price. Update the FetchProductData
query below to fetch more fields as required (list of fields here).
import { gql, useLazyQuery } from "@apollo/client";
const FETCH_PRODUCT_DATA = gql`
query FetchProductData($input: ProductByIDInput!) {
productByID(input: $input) {
marketplace
title
vendor
price {
displayValue
}
images {
url
}
variants {
title
}
}
}
`;
const [fetchProduct, { data: fetchProductData, loading: fetchProductLoading }] = useLazyQuery(FETCH_PRODUCT_DATA);
const onFetchProduct = () => {
const input = {
id: productId,
marketplace: <<SHOPIFY / AMAZON>>,
};
fetchProduct({ variables: { input }
Note: Now that the products are in Rye’s inventory, how should you manage access to these products? There are two solutions:
- Create a cron job which callsproductByID
every 12 hours and add products to your database
- Call theproductByID
API real-time in the client without the need for a database of products
Now that the product is in Rye’s inventory, you can fetch product information via the productByID
query. This allows you to showcase products and display accurate, up-to-date information, and allows users to make informed purchasing decisions.
Input:
The product ID
The marketplace of the product ('Amazon' or 'Shopify')
Output:
All the requested product data
The code snippet below only fetches specific product data including title, vendor, variants, and price. Update the FetchProductData
query below to fetch more fields as required (list of fields here).
import { gql, useLazyQuery } from "@apollo/client";
const FETCH_PRODUCT_DATA = gql`
query FetchProductData($input: ProductByIDInput!) {
productByID(input: $input) {
marketplace
title
vendor
price {
displayValue
}
images {
url
}
variants {
title
}
}
}
`;
const [fetchProduct, { data: fetchProductData, loading: fetchProductLoading }] = useLazyQuery(FETCH_PRODUCT_DATA);
const onFetchProduct = () => {
const input = {
id: productId,
marketplace: <<SHOPIFY / AMAZON>>,
};
fetchProduct({ variables: { input }
Note: Now that the products are in Rye’s inventory, how should you manage access to these products? There are two solutions:
- Create a cron job which callsproductByID
every 12 hours and add products to your database
- Call theproductByID
API real-time in the client without the need for a database of products
Now that the product is in Rye’s inventory, you can fetch product information via the productByID
query. This allows you to showcase products and display accurate, up-to-date information, and allows users to make informed purchasing decisions.
Input:
The product ID
The marketplace of the product ('Amazon' or 'Shopify')
Output:
All the requested product data
The code snippet below only fetches specific product data including title, vendor, variants, and price. Update the FetchProductData
query below to fetch more fields as required (list of fields here).
import { gql, useLazyQuery } from "@apollo/client";
const FETCH_PRODUCT_DATA = gql`
query FetchProductData($input: ProductByIDInput!) {
productByID(input: $input) {
marketplace
title
vendor
price {
displayValue
}
images {
url
}
variants {
title
}
}
}
`;
const [fetchProduct, { data: fetchProductData, loading: fetchProductLoading }] = useLazyQuery(FETCH_PRODUCT_DATA);
const onFetchProduct = () => {
const input = {
id: productId,
marketplace: <<SHOPIFY / AMAZON>>,
};
fetchProduct({ variables: { input }
Note: Now that the products are in Rye’s inventory, how should you manage access to these products? There are two solutions:
- Create a cron job which callsproductByID
every 12 hours and add products to your database
- Call theproductByID
API real-time in the client without the need for a database of products
Step 3: Create a cart with an item for user
Create a cart with an item via the createCart
mutation.
Rye offers the ability to add multiple products to your cart from multiple different stores!
Note: For Shopify products, we add items to cart via the
variantId
and NOTproductId
Input:
The cart ID
An array of objects which have the quantity and the product ID
Output:
Cart data
import { gql, useMutation } from "@apollo/client";
export const CREATE_CART = gql`
mutation createCart($input: CartCreateInput!) {
createCart(input: $input) {
cart {
id
}
}
}
`;
const [createCart, { data: createCartData, loading: createCartLoading }] = useMutation(CREATE_CART);
const onCartCreate = () => {
const input = {
"input": {
"items": {
"amazonCartItemsInput": [{
"quantity": 1,
"productId": "B0B1H2MDCX"
}]
}
}
}
createCart({ variables: { input }})
};
Create a cart with an item via the createCart
mutation.
Rye offers the ability to add multiple products to your cart from multiple different stores!
Note: For Shopify products, we add items to cart via the
variantId
and NOTproductId
Input:
The cart ID
An array of objects which have the quantity and the product ID
Output:
Cart data
import { gql, useMutation } from "@apollo/client";
export const CREATE_CART = gql`
mutation createCart($input: CartCreateInput!) {
createCart(input: $input) {
cart {
id
}
}
}
`;
const [createCart, { data: createCartData, loading: createCartLoading }] = useMutation(CREATE_CART);
const onCartCreate = () => {
const input = {
"input": {
"items": {
"amazonCartItemsInput": [{
"quantity": 1,
"productId": "B0B1H2MDCX"
}]
}
}
}
createCart({ variables: { input }})
};
Create a cart with an item via the createCart
mutation.
Rye offers the ability to add multiple products to your cart from multiple different stores!
Note: For Shopify products, we add items to cart via the
variantId
and NOTproductId
Input:
The cart ID
An array of objects which have the quantity and the product ID
Output:
Cart data
import { gql, useMutation } from "@apollo/client";
export const CREATE_CART = gql`
mutation createCart($input: CartCreateInput!) {
createCart(input: $input) {
cart {
id
}
}
}
`;
const [createCart, { data: createCartData, loading: createCartLoading }] = useMutation(CREATE_CART);
const onCartCreate = () => {
const input = {
"input": {
"items": {
"amazonCartItemsInput": [{
"quantity": 1,
"productId": "B0B1H2MDCX"
}]
}
}
}
createCart({ variables: { input }})
};
Step 4: Update buyer identity for the cart
Now that you have a cart which is ready to be checked out, the next step is to get the users details and shipping address. A shipping address is required for us to get shipping methods in the next step.
To associate a cart with a buyer identity, we use the updateCartBuyerIdentity
mutation. This mutation deals with the gnarly address validation issues, ensuring that you will only be able to enter valid information to proceed.
Input:
The cart ID
User shipping address information
Output:
Cart data
import { gql, useMutation } from "@apollo/client";
export const UPDATE_BUYER_IDENTITY = gql`
mutation updateCartBuyerIdentity($input: CartBuyerIdentityUpdateInput!) {
updateCartBuyerIdentity(input: $input) {
cart {
id
stores {
... on ShopifyStore {
errors {
code
message
details {
variantIds
}
}
store
cartLines {
quantity
variant {
id
}
}
offer {
subtotal {
value
displayValue
currency
}
shippingMethods {
id
label
price {
value
displayValue
currency
}
taxes {
value
displayValue
currency
}
total {
value
displayValue
currency
}
}
}
}
}
}
}
}
`;
const [updateBuyerIdentity, { data: updateBuyerIdentityResult, loading: updateBuyerIdentityLoading }] = useMutation(UPDATE_BUYER_IDENTITY);
const onSubmitCartInformation = () => {
const input = {
id: cartId,
buyerIdentity: {
city,
address1,
address2,
firstName,
lastName,
email,
phone,
postalCode: zip,
provinceCode,
countryCode: country,
},
};
updateBuyerIdentity({ variables: { input }})
};
Now that you have a cart which is ready to be checked out, the next step is to get the users details and shipping address. A shipping address is required for us to get shipping methods in the next step.
To associate a cart with a buyer identity, we use the updateCartBuyerIdentity
mutation. This mutation deals with the gnarly address validation issues, ensuring that you will only be able to enter valid information to proceed.
Input:
The cart ID
User shipping address information
Output:
Cart data
import { gql, useMutation } from "@apollo/client";
export const UPDATE_BUYER_IDENTITY = gql`
mutation updateCartBuyerIdentity($input: CartBuyerIdentityUpdateInput!) {
updateCartBuyerIdentity(input: $input) {
cart {
id
stores {
... on ShopifyStore {
errors {
code
message
details {
variantIds
}
}
store
cartLines {
quantity
variant {
id
}
}
offer {
subtotal {
value
displayValue
currency
}
shippingMethods {
id
label
price {
value
displayValue
currency
}
taxes {
value
displayValue
currency
}
total {
value
displayValue
currency
}
}
}
}
}
}
}
}
`;
const [updateBuyerIdentity, { data: updateBuyerIdentityResult, loading: updateBuyerIdentityLoading }] = useMutation(UPDATE_BUYER_IDENTITY);
const onSubmitCartInformation = () => {
const input = {
id: cartId,
buyerIdentity: {
city,
address1,
address2,
firstName,
lastName,
email,
phone,
postalCode: zip,
provinceCode,
countryCode: country,
},
};
updateBuyerIdentity({ variables: { input }})
};
Now that you have a cart which is ready to be checked out, the next step is to get the users details and shipping address. A shipping address is required for us to get shipping methods in the next step.
To associate a cart with a buyer identity, we use the updateCartBuyerIdentity
mutation. This mutation deals with the gnarly address validation issues, ensuring that you will only be able to enter valid information to proceed.
Input:
The cart ID
User shipping address information
Output:
Cart data
import { gql, useMutation } from "@apollo/client";
export const UPDATE_BUYER_IDENTITY = gql`
mutation updateCartBuyerIdentity($input: CartBuyerIdentityUpdateInput!) {
updateCartBuyerIdentity(input: $input) {
cart {
id
stores {
... on ShopifyStore {
errors {
code
message
details {
variantIds
}
}
store
cartLines {
quantity
variant {
id
}
}
offer {
subtotal {
value
displayValue
currency
}
shippingMethods {
id
label
price {
value
displayValue
currency
}
taxes {
value
displayValue
currency
}
total {
value
displayValue
currency
}
}
}
}
}
}
}
}
`;
const [updateBuyerIdentity, { data: updateBuyerIdentityResult, loading: updateBuyerIdentityLoading }] = useMutation(UPDATE_BUYER_IDENTITY);
const onSubmitCartInformation = () => {
const input = {
id: cartId,
buyerIdentity: {
city,
address1,
address2,
firstName,
lastName,
email,
phone,
postalCode: zip,
provinceCode,
countryCode: country,
},
};
updateBuyerIdentity({ variables: { input }})
};
Step 5: Select a shipping method for the cart
Now that the cart has been associated with a buyer, Rye fetches all the possible shipping options. If a cart has products from more than 1 store, Rye sends possible shipping options for each store.
To select a shipping option for a store, we can use the updateCartSelectedShippingOptions
mutation.
Please ensure to select shipping options for each store in your cart.
Input:
The cart ID
An array of objects which have the shipping ID and the store name
Output:
Cart data
import { gql, useMutation } from "@apollo/client";
export const SELECT_SHIPPING_METHOD = gql`
mutation ($input: UpdateCartSelectedShippingOptionsInput!) {
updateCartSelectedShippingOptions(input: $input) {
cart {
id
stores {
... on ShopifyStore {
store
offer {
selectedShippingMethod {
id
label
price {
displayValue
value
currency
}
taxes {
displayValue
value
currency
}
total {
displayValue
value
currency
}
}
}
}
}
}
}
}
`;
const [selectShippingMethod, { data: selectShippingMethodData, loading: selectShippingMethodLoading }] = useMutation(SELECT_SHIPPING_METHOD);
const input = {
id: cartId,
shippingOptions: [
{
store: store,
shippingId: shippingId
}
]
};
selectShippingMethod({ variables: { input }})
};
Now that the cart has been associated with a buyer, Rye fetches all the possible shipping options. If a cart has products from more than 1 store, Rye sends possible shipping options for each store.
To select a shipping option for a store, we can use the updateCartSelectedShippingOptions
mutation.
Please ensure to select shipping options for each store in your cart.
Input:
The cart ID
An array of objects which have the shipping ID and the store name
Output:
Cart data
import { gql, useMutation } from "@apollo/client";
export const SELECT_SHIPPING_METHOD = gql`
mutation ($input: UpdateCartSelectedShippingOptionsInput!) {
updateCartSelectedShippingOptions(input: $input) {
cart {
id
stores {
... on ShopifyStore {
store
offer {
selectedShippingMethod {
id
label
price {
displayValue
value
currency
}
taxes {
displayValue
value
currency
}
total {
displayValue
value
currency
}
}
}
}
}
}
}
}
`;
const [selectShippingMethod, { data: selectShippingMethodData, loading: selectShippingMethodLoading }] = useMutation(SELECT_SHIPPING_METHOD);
const input = {
id: cartId,
shippingOptions: [
{
store: store,
shippingId: shippingId
}
]
};
selectShippingMethod({ variables: { input }})
};
Now that the cart has been associated with a buyer, Rye fetches all the possible shipping options. If a cart has products from more than 1 store, Rye sends possible shipping options for each store.
To select a shipping option for a store, we can use the updateCartSelectedShippingOptions
mutation.
Please ensure to select shipping options for each store in your cart.
Input:
The cart ID
An array of objects which have the shipping ID and the store name
Output:
Cart data
import { gql, useMutation } from "@apollo/client";
export const SELECT_SHIPPING_METHOD = gql`
mutation ($input: UpdateCartSelectedShippingOptionsInput!) {
updateCartSelectedShippingOptions(input: $input) {
cart {
id
stores {
... on ShopifyStore {
store
offer {
selectedShippingMethod {
id
label
price {
displayValue
value
currency
}
taxes {
displayValue
value
currency
}
total {
displayValue
value
currency
}
}
}
}
}
}
}
}
`;
const [selectShippingMethod, { data: selectShippingMethodData, loading: selectShippingMethodLoading }] = useMutation(SELECT_SHIPPING_METHOD);
const input = {
id: cartId,
shippingOptions: [
{
store: store,
shippingId: shippingId
}
]
};
selectShippingMethod({ variables: { input }})
};
Step 6: Payment + submit cart
Now that we have a cart with items, an associated buyer identity, and a selected shipping method, we are ready to checkout!
To submit the cart, Rye provides two approaches:
Rye Pay (link for docs) (npm package link)
submitCart
mutation (link for docs)
We are using Rye Pay for simplicity.
For testing this with a test card:
• Ensure that an item from a test store has been added (for example: try adding variant43683179954478
to your cart for a test product from a test store in Step 5)
• Use the card4242424242424242
with any first name, last name, 3 digit CVV, and an expiry date in the future
Input:
The cart ID
Billing address information
CC details (in the Spreedly fields)
Output:
Order submission status
import { RyePay } from "@rye-api/rye-pay";
const ryePay = new RyePay();
const apiKey = "x";
const loadRyePay = () => {
ryePay.init({
apiKey,
environment: 'prod',
cvvEl: 'payment-cvv',
numberEl: 'payment-number',
onReady: () => {
ryePay.setValue('number', 4242424242424242);
ryePay.setValue('cvv', 123);
},
onErrors: (errors) => {
for (const { key, message, attribute } of errors) {
alert(`new error: ${key}-${message}-${attribute}`)
}
},
enableLogging: true,
onIFrameError: (err) => {
console.log(`frameError: ${JSON.stringify(err)}`);
},
onCartSubmitted: (result) => {
setLoadingResult(false);
},
});
};
loadRyePay();
}, []);
const submit = () => {
ryePay.submit({
first_name,
last_name,
month,
year,
cartId,
address1,
address2,
zip,
city,
country,
state,
phone_number,
shopperIp: '<YOUR IP>',
});
setLoadingResult(true);
};
Now that we have a cart with items, an associated buyer identity, and a selected shipping method, we are ready to checkout!
To submit the cart, Rye provides two approaches:
Rye Pay (link for docs) (npm package link)
submitCart
mutation (link for docs)
We are using Rye Pay for simplicity.
For testing this with a test card:
• Ensure that an item from a test store has been added (for example: try adding variant43683179954478
to your cart for a test product from a test store in Step 5)
• Use the card4242424242424242
with any first name, last name, 3 digit CVV, and an expiry date in the future
Input:
The cart ID
Billing address information
CC details (in the Spreedly fields)
Output:
Order submission status
import { RyePay } from "@rye-api/rye-pay";
const ryePay = new RyePay();
const apiKey = "x";
const loadRyePay = () => {
ryePay.init({
apiKey,
environment: 'prod',
cvvEl: 'payment-cvv',
numberEl: 'payment-number',
onReady: () => {
ryePay.setValue('number', 4242424242424242);
ryePay.setValue('cvv', 123);
},
onErrors: (errors) => {
for (const { key, message, attribute } of errors) {
alert(`new error: ${key}-${message}-${attribute}`)
}
},
enableLogging: true,
onIFrameError: (err) => {
console.log(`frameError: ${JSON.stringify(err)}`);
},
onCartSubmitted: (result) => {
setLoadingResult(false);
},
});
};
loadRyePay();
}, []);
const submit = () => {
ryePay.submit({
first_name,
last_name,
month,
year,
cartId,
address1,
address2,
zip,
city,
country,
state,
phone_number,
shopperIp: '<YOUR IP>',
});
setLoadingResult(true);
};
Now that we have a cart with items, an associated buyer identity, and a selected shipping method, we are ready to checkout!
To submit the cart, Rye provides two approaches:
Rye Pay (link for docs) (npm package link)
submitCart
mutation (link for docs)
We are using Rye Pay for simplicity.
For testing this with a test card:
• Ensure that an item from a test store has been added (for example: try adding variant43683179954478
to your cart for a test product from a test store in Step 5)
• Use the card4242424242424242
with any first name, last name, 3 digit CVV, and an expiry date in the future
Input:
The cart ID
Billing address information
CC details (in the Spreedly fields)
Output:
Order submission status
import { RyePay } from "@rye-api/rye-pay";
const ryePay = new RyePay();
const apiKey = "x";
const loadRyePay = () => {
ryePay.init({
apiKey,
environment: 'prod',
cvvEl: 'payment-cvv',
numberEl: 'payment-number',
onReady: () => {
ryePay.setValue('number', 4242424242424242);
ryePay.setValue('cvv', 123);
},
onErrors: (errors) => {
for (const { key, message, attribute } of errors) {
alert(`new error: ${key}-${message}-${attribute}`)
}
},
enableLogging: true,
onIFrameError: (err) => {
console.log(`frameError: ${JSON.stringify(err)}`);
},
onCartSubmitted: (result) => {
setLoadingResult(false);
},
});
};
loadRyePay();
}, []);
const submit = () => {
ryePay.submit({
first_name,
last_name,
month,
year,
cartId,
address1,
address2,
zip,
city,
country,
state,
phone_number,
shopperIp: '<YOUR IP>',
});
setLoadingResult(true);
};
Step 7: Post-checkout experience
Once an order has been submitted, Rye sends you the following web-hooks/push-notifications to ensure that you have complete visibility in the progress of the order:
Order submission:
Order submission started
Order submission succeeded
Order placed / Order failed
Payment:
Payment succeeded
Payment failed
Payment refunded
Tracking webhooks (For individual shipments)
Order cancellation:
Order cancel started
Order cancel succeeded
Order cancel failed
For more details on web-hooks available: https://docs.rye.com/docs/webhook-updates
To configure your web-hooks:
Head over to https://console.rye.com/account
Enter a valid callback endpoint for your backend under the "Webhook section", and click save!
Once an order has been submitted, Rye sends you the following web-hooks/push-notifications to ensure that you have complete visibility in the progress of the order:
Order submission:
Order submission started
Order submission succeeded
Order placed / Order failed
Payment:
Payment succeeded
Payment failed
Payment refunded
Tracking webhooks (For individual shipments)
Order cancellation:
Order cancel started
Order cancel succeeded
Order cancel failed
For more details on web-hooks available: https://docs.rye.com/docs/webhook-updates
To configure your web-hooks:
Head over to https://console.rye.com/account
Enter a valid callback endpoint for your backend under the "Webhook section", and click save!
Once an order has been submitted, Rye sends you the following web-hooks/push-notifications to ensure that you have complete visibility in the progress of the order:
Order submission:
Order submission started
Order submission succeeded
Order placed / Order failed
Payment:
Payment succeeded
Payment failed
Payment refunded
Tracking webhooks (For individual shipments)
Order cancellation:
Order cancel started
Order cancel succeeded
Order cancel failed
For more details on web-hooks available: https://docs.rye.com/docs/webhook-updates
To configure your web-hooks:
Head over to https://console.rye.com/account
Enter a valid callback endpoint for your backend under the "Webhook section", and click save!
That's it!
In 7 simple steps, you have built an end-to-end eComm experience for your social platform using Rye APIs.
The best part? These APIs are GQL queries and mutations that can be configured for any codebase and tech-stack, giving power back to developers. Our APIs are free to use. So keep testing and iterating on what we’ve built so far!
We love building the future of eComm and hearing your big ideas. Get in touch to see how we can help you scale your platform together.
In 7 simple steps, you have built an end-to-end eComm experience for your social platform using Rye APIs.
The best part? These APIs are GQL queries and mutations that can be configured for any codebase and tech-stack, giving power back to developers. Our APIs are free to use. So keep testing and iterating on what we’ve built so far!
We love building the future of eComm and hearing your big ideas. Get in touch to see how we can help you scale your platform together.
In 7 simple steps, you have built an end-to-end eComm experience for your social platform using Rye APIs.
The best part? These APIs are GQL queries and mutations that can be configured for any codebase and tech-stack, giving power back to developers. Our APIs are free to use. So keep testing and iterating on what we’ve built so far!
We love building the future of eComm and hearing your big ideas. Get in touch to see how we can help you scale your platform together.
Related articles
Related articles
Learn How to Build with Rye
Product
Resources